Script component
The Script component allows you to modify messages using scripts written in JavaScript or Groovy. The scripts can either be provided directly or uploaded as files. Scripts are able to read and modify the body, headers, and properties of messages that pass through the component.
To simplify development of scripts, the Script component offers the ability to evaluate scripts given a set of test values for the body, headers, and properties of a message.
Configuration
The Script component has the following configuration options:
Property | Description |
---|---|
Script | The script that should be evaluated for each message passing through the component. |
File Upload | The script that should be evaluated for each message in the form of a file. |
Language | The language used for the script. |
In Headers | A list of comma-separated name-value pairs representing headers for testing purposes. The names and values should be quoted. |
In Properties | A list of comma-separated name-value pairs representing properties for testing purposes. The names and values should be quoted. |
In Body | A body for testing purposes |
Out Headers | The list of headers after evaluating the script. |
Out Properties | The list of properties after evaluating the script. |
Out Body | The body of the message after evaluation. |
Examples
Below there are examples of what can be done in the script component. Also see the Using time in Dovetail guide for more Groovy script examples.
Working with headers
// Get header values
var headerAsIs = request.getHeader("myHeader");
var headerAsString = request.getHeader("myHeader", String.class);
// Set new or overwrite existing header
request.setHeader("newHeader", "header value");
// Remove headers
request.removeHeader("headerName");
// Get header values
def headerAsIs = request.getHeader("myHeader");
def headerAsString = request.getHeader("myHeader", String.class);
// Set new or overwrite existing header
request.setHeader("newHeader", "header value");
// Remove headers
request.removeHeader("headerName");
Working with the body
// Get the body from the message
var bodyAsIs = request.body;
var bodyAsString = request.getBody(String.class); // Other Java classes can also be used
// Setting the body of the message
result = "My new body"
// Set this at the end of a script to prevent the message body becoming null
result = request.body;
// Get the body from the message
def bodyAsIs = request.body;
def bodyAsString = request.getBody(String.class); // Other Java classes can also be used
// Setting the body of the message
result = "My new body"
// Set this at the end of a script to prevent the message body becoming null
result = request.body;
Import third party libraries in JavaScript scripts
By using the load
function third party libraries can be imported and used in
JavaScript scripts:
load(
"https://cdnjs.cloudflare.com/ajax/libs/crypto-js/3.1.9-1/crypto-js.min.js",
"https://cdnjs.cloudflare.com/ajax/libs/crypto-js/3.1.9-1/hmac-sha256.min.js",
"https://cdnjs.cloudflare.com/ajax/libs/crypto-js/3.1.9-1/enc-base64.min.js"
);
Full example with script evaluation
The image below shows the result of evaluating a script for a given body, set of headers and set of properties.
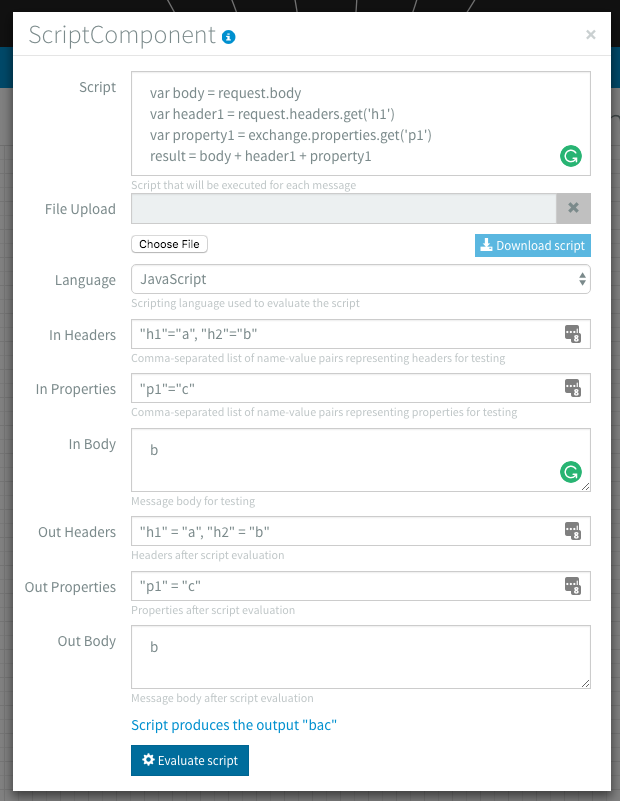
Remarks
- The JavaScript that you can use in the script component is not the same
implementation as in web browsers. It is an open source implementation of
JavaScript for Java. We advice that you use standard JavaScript syntax also known
as
ES5
. Newer syntax and other features are not supported at the moment. - The Groovy version that is used in the script component is
2.4.15
. - In the flow designer you enter test data in text inputs. If those text inputs are empty,
the script is actually tested with empty strings. An empty text input is therefore
not considered
null
. If the flow is running and the body is empty, it will benull
and not""
. If you convert this empty body to string you will get"null"
. It is best to test your script withnull
for theIn body
.